C# ASP.NET Developer Roadmap
Naveen Singh
22 days ago
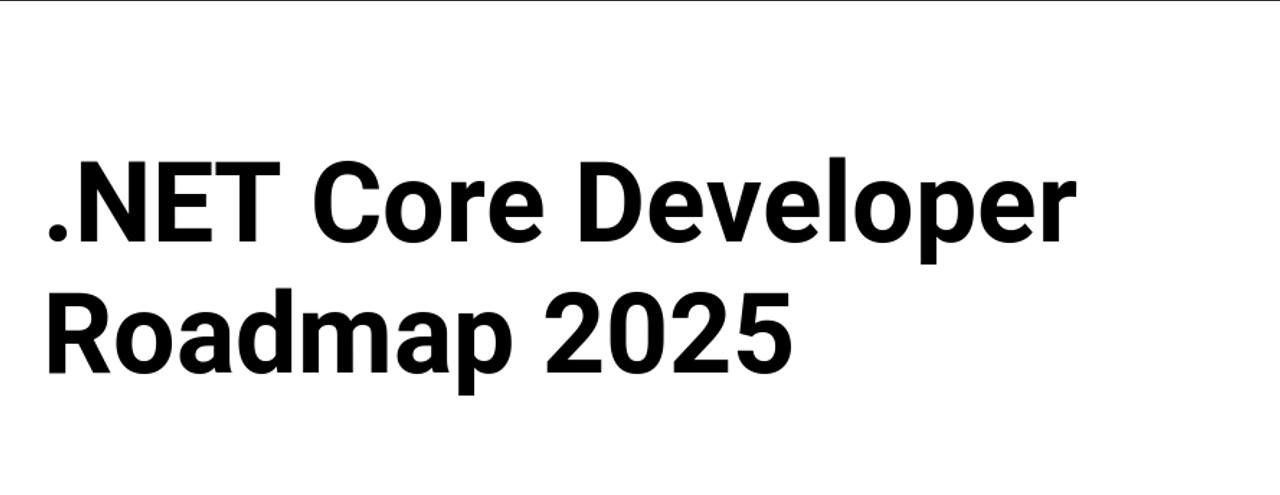
🔴 C# ASP.NET Developer Roadmap
Introduction
Becoming a proficient C# ASP.NET Developer requires a well-planned learning path that covers both foundational concepts and advanced topics. Whether you're a beginner or leveling up your skills, this roadmap will guide you step-by-step through everything you need to master the .NET ecosystem and modern web development with ASP.NET Core.
🔵 Basics
🟢 C# Fundamentals
Every .NET developer should start with the fundamentals of C#. This includes:
- Data Types and Variables
- Control Flow (Loops, Conditions)
- Functions and Methods
- Object-Oriented Programming (OOP)
- Collections like List, Dictionary, etc.
- Exception Handling
- LINQ (Language Integrated Query) for data manipulation
🟢 .NET Basics
Understanding the core of the .NET ecosystem:
- .NET Runtime and Common Language Runtime (CLR)
- NuGet Package Manager for managing libraries
- Assemblies and DLLs
- Garbage Collection
🔵 Version Control
🟢 Git
Version control is essential for collaboration:
- Basic Git commands (clone, commit, push, pull)
- Branching and Merging workflows
- Using platforms like GitHub or GitLab
🔵 Package Managers
🟢 NuGet
- Learn how to manage and integrate third-party libraries via NuGet.
🔵 Web Development with ASP.NET Core
🟢 ASP.NET Core Basics
- Middleware & Request Pipeline
- Routing & Endpoints
- Dependency Injection
- Controllers & Actions
- Model Binding & Validation
🟢 Razor Pages & MVC
- Views, Partial Views, and Layouts
- View Components
- Tag Helpers & View Models
🟢 Blazor (Optional)
- Blazor Server vs WebAssembly
- Building interactive UI components
- State management with Blazor
🔵 APIs and Microservices
🟢 RESTful APIs
- Creating APIs with Controllers and Routes
- HTTP Methods & Status Codes
- API Validation
- API Versioning
- API documentation with Swagger / OpenAPI
🟢 Authentication & Authorization
- Implementing JWT (JSON Web Tokens)
- OAuth2 & OpenID Connect flows
- IdentityServer integration
🟢 Real-time Communication
- SignalR for WebSockets and real-time apps
- gRPC for high-performance communication
🟢 Microservices Architecture
- API Gateway pattern
- Service Discovery tools
- Event-Driven Architecture using message brokers
🔵 Databases & ORM
🟢 Relational Databases
- SQL Server, PostgreSQL, MySQL
🟢 Entity Framework Core
- Code First & Database First approaches
- Migrations & Data Seeding
- Querying data with LINQ
- Transactions and Unit of Work pattern
- EF Core performance tuning
🔵 Testing
🟢 Unit Testing
- Frameworks like xUnit, MSTest, NUnit
🟢 Integration Testing
- End-to-end testing strategies
🟢 API Testing
- Tools like Postman & RestSharp
🟢 Mocking Dependencies
- Libraries like Moq & FakeItEasy
🔵 Performance Optimization
- Caching (MemoryCache, Redis)
- Async/Await & Multithreading concepts
- Connection Pooling
- Avoiding and detecting memory leaks
🔵 Cloud & Deployment
- Hosting on Azure, AWS, or Google Cloud
- CI/CD pipelines (GitHub Actions, Azure DevOps)
- Docker & Kubernetes for containerization
- Serverless architectures with Azure Functions
🔵 Security Best Practices
- Data Protection (Encryption, Hashing)
- Securing APIs (Rate Limiting, CORS, Authentication)
- Mitigating OWASP Top 10 vulnerabilities
🔵 Advanced Topics
- Clean Architecture for scalable solutions
- CQRS & MediatR pattern for separation of concerns
- Event-Driven Systems with RabbitMQ or Kafka
- GraphQL APIs with ASP.NET Core
🔵 Soft Skills
- Effective communication & writing documentation
- Code Reviews & Pair Programming
- Strong Debugging & Problem-solving mindset
Final Thoughts
This roadmap is a blueprint for becoming a complete C# ASP.NET Core Developer. Whether you're building microservices, high-performance APIs, or modern web apps with Blazor, this journey will equip you with all the essential tools and concepts.
Stay consistent, keep building, and follow best practices!
Powered by Froala Editor