Why FirstOrDefault Should No Longer Be Used
Naveen Singh
24 days ago
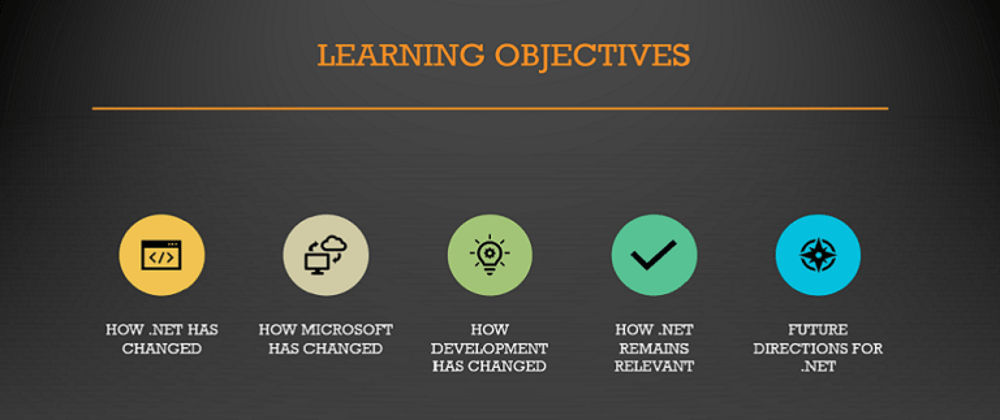
FirstOrDefault()
is commonly used in LINQ (Language Integrated Query) in .NET to retrieve the first element of a sequence, or the default value (usually null
for reference types or default(T)
for value types) if the sequence is empty.
Reasons to reconsider using FirstOrDefault
:
- Unclear Intent: The name
FirstOrDefault
can lead to misunderstanding about the logic. It's not always clear whether the default value (null
) is expected or signifies an error condition. - Nullability Issues: Using
FirstOrDefault
can unintentionally lead toNullReferenceException
because it can returnnull
when no matching element is found. If the code doesn't explicitly handlenull
, it could cause runtime exceptions. - Performance: While
FirstOrDefault
works for most cases, when you're certain the collection contains at least one element, usingFirst()
(which throws an exception when no element is found) is more appropriate. It also helps make your code more defensive and catches potential errors earlier. - Improved Code Readability: Newer C# versions and patterns encourage better handling of nullability with features like nullable reference types (
T?
) and pattern matching, making the use ofFirstOrDefault
less necessary and promoting more expressive alternatives.
Alternatives to FirstOrDefault
Use First()
:
If you're certain that there is at least one element and you want to ensure an exception is thrown if there isn't, First()
is a more appropriate choice. This enforces the assumption and avoids silently passing null
.
var firstItem = list.First(); // Throws an exception if the list is empty
Use SingleOrDefault()
:
If you're expecting only one item but want to ensure that no more than one exists, SingleOrDefault()
is more strict. It throws an exception if more than one item is found.
var singleItem = list.SingleOrDefault(); // Ensures only one item, returns default if empty
Explicit Null Checks:
Instead of using FirstOrDefault
and risking null
, consider explicitly handling empty cases upfront with Any()
or Count()
, which can make your intent clearer:
if (list.Any())
{
var firstItem = list.First();
}
else
{
// Handle empty list case
}
Provenance of FirstOrDefault
FirstOrDefault()
is part of the LINQ (Language Integrated Query) extensions in C#. It is widely used in querying sequences such as IEnumerable<T>
. It was introduced as part of .NET 3.5 when LINQ was added to the language, aimed at providing more declarative and readable ways to query collections.
How FirstOrDefault
Is Used
FirstOrDefault()
is typically used to retrieve the first element of a collection or return a default value if the collection is empty or no matching element is found. Here's how it is typically used:
TSource FirstOrDefault<TSource>(this IEnumerable<TSource> source);
Example of Usage:
var numbers = new List<int> { 1, 2, 3 };
var firstNumber = numbers.FirstOrDefault();
var emptyList = new List<int>();
var defaultNumber = emptyList.FirstOrDefault();
The method is particularly useful when querying collections where the result may be empty, and returning null
or default(T)
is acceptable.
Comparison Examples
Example 1: FirstOrDefault
Returning Default Value
var people = new List<Person> { new Person("John"), new Person("Jane") };
Person person = people.FirstOrDefault(p => p.Name == "Bob");
// person will be null because "Bob" doesn't exist in the list
In this case, FirstOrDefault
can cause a NullReferenceException
if you attempt to access properties of person
without null checking.
Example 2: Safer Approach Using First
with Null Handling
var people = new List<Person> { new Person("John"), new Person("Jane") };
if (people.Any(p => p.Name == "Bob"))
{
Person person = people.First(p => p.Name == "Bob");
// Do something with person
}
else
{
// Handle case where "Bob" is not found
}
This approach avoids the ambiguity of returning null
by explicitly checking if a person named "Bob" exists and handling the empty case more safely.
Example 3: Using First
for More Defensive Code
var numbers = new List<int> { 1, 2, 3 };
var firstNumber = numbers.First();
// Will throw an exception if the list is empty
This method throws an exception if the collection is empty, which can help identify logic errors earlier in development, rather than allowing the code to continue with potentially incorrect assumptions.
Conclusion
While FirstOrDefault()
is useful in certain scenarios, it is becoming less preferred in modern C# development due to its potential for causing unintended null
values and reduced code clarity. Alternatives like First()
, SingleOrDefault()
, or explicit null handling via Any()
or Count()
can make code more robust, readable, and error-resistant.
Powered by Froala Editor